ここでは、FirebaseのNoSQLデータベース(Cloud Firestore)を利用するための初期設定について解説します。
FlutterでFirestoreを利用できるようにするため、pub.devからcloud_firestore
プラグインをインストールします。
https://pub.dev/packages/cloud_firestore/
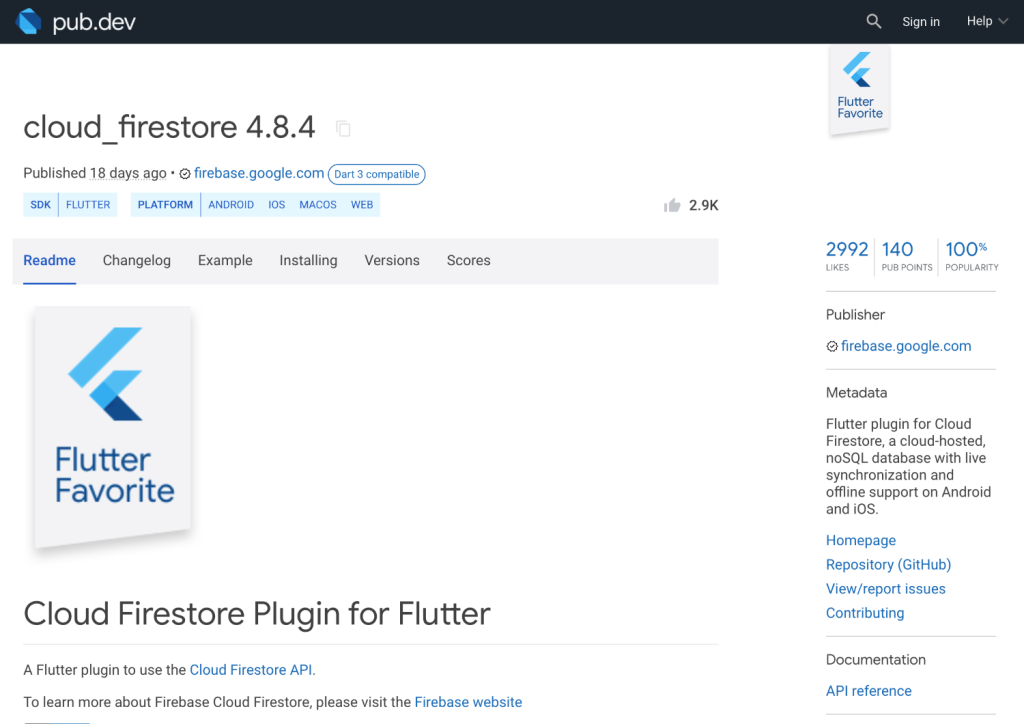
AndroidStudioのターミナルから下記コマンドを実行すると、pubspec.yamlにcloud_firestoreが追加されインストールされます。
flutter pub add cloud_firestore
dependencies:
flutter:
sdk: flutter
# The following adds the Cupertino Icons font to your application.
# Use with the CupertinoIcons class for iOS style icons.
cupertino_icons: ^1.0.2
firebase_core: ^2.15.0
cloud_firestore: ^4.8.4
iOSのビルドにおいてcloud_firestoreは、ビルド処理に時間が掛かります。そのため予めコンパイル済みのフレームワークを利用するとビルド時間を短縮できます。Apple M2チップで試すと5分30秒から25秒程度まで短縮されました。なおAndroidでは、20秒から4秒程度になりました。
コンパイル済みのcloud_firestoreを利用するには、/ios/Podfile
ファイルを開きtarget 'Runner' do
の部分にコードを追加します。
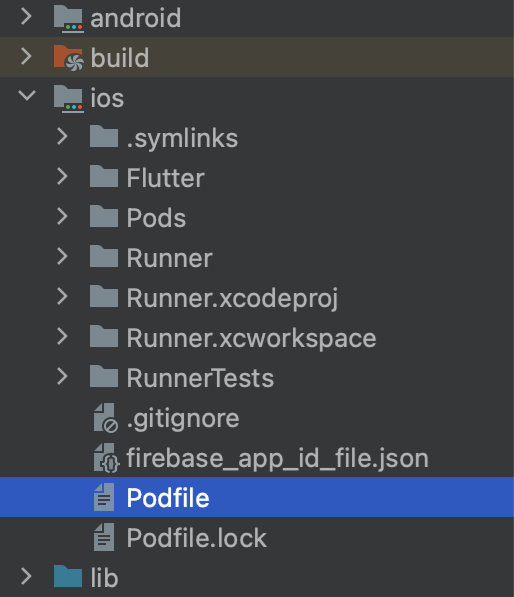
/ios/Podfile
pod 'FirebaseFirestore', :git => 'https://github.com/invertase/firestore-ios-sdk-frameworks.git', :tag => '10.12.0'
(変更前)
target 'Runner' do
use_frameworks!
use_modular_headers!
flutter_install_all_ios_pods File.dirname(File.realpath(__FILE__))
target 'RunnerTests' do
inherit! :search_paths
end
end
(変更後)
target 'Runner' do
pod 'FirebaseFirestore', :git => 'https://github.com/invertase/firestore-ios-sdk-frameworks.git', :tag => '10.12.0'
use_frameworks!
use_modular_headers!
flutter_install_all_ios_pods File.dirname(File.realpath(__FILE__))
target 'RunnerTests' do
inherit! :search_paths
end
end
また、cocospodのバージョンは、1.9.1以降である必要があります。pod --version
で調べることができます。
pod --version
1.12.1
公式サイトの解説では、追加コードでのバージョン指定がtag => '8.15.0'
となっています。追加コードを記述する前に、どのくらい時間が掛かるか通常のビルドを試すと8.15.0
はすでに古いバージョンであるため、より高いバージョンが指定されてビルドされます。
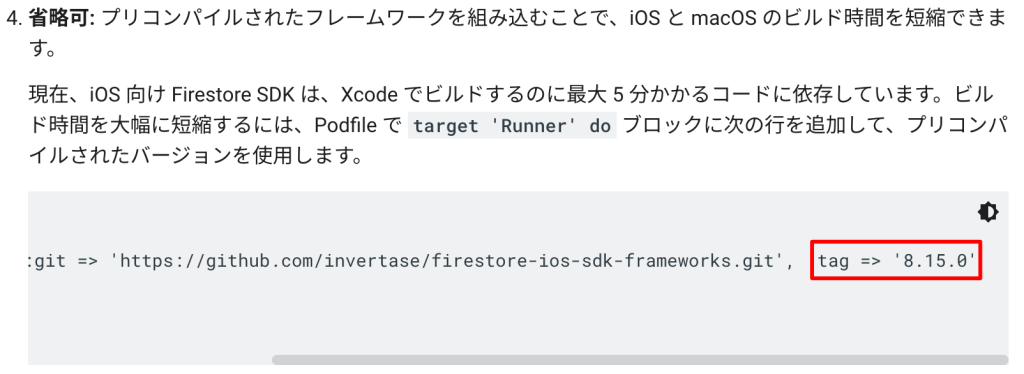
GitHubのfirestore-ios-sdk-frameworksを確認すると、8.15.0より高いバージョンが多くあることが分かります。公式の内容は、記載された当時のバージョンのままアップデートされず残っているものと思われます。
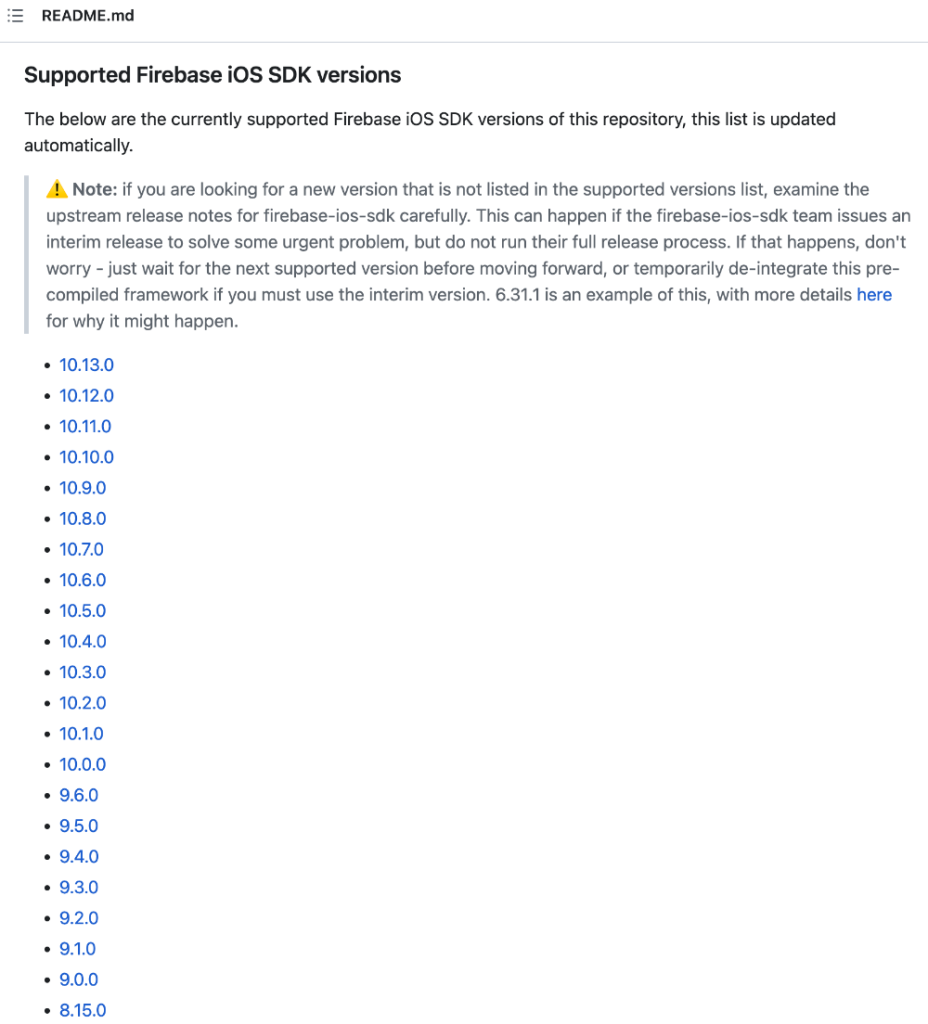
そのため、8.15.0
より高いバージョンで(10.12.0
)一度ビルドされた後に、追加コードを記述すると、Podfile.lock
には10.12.0
が存在して、8.15.0
を指定すると整合が取れなくなり下記のようなエラーが発生します。8.15.0
を使用する場合は、一度Podfile.lockの削除を行います。
CocoaPods' output:
↳
Preparing
Analyzing dependencies
Inspecting targets to integrate
Using `ARCHS` setting to build architectures of target `Pods-Runner`: (``)
Using `ARCHS` setting to build architectures of target `Pods-RunnerTests`: (``)
Finding Podfile changes
A FirebaseFirestore
- Flutter
- cloud_firestore
- firebase_core
Fetching external sources
-> Pre-downloading: `FirebaseFirestore` from `https://github.com/invertase/firestore-ios-sdk-frameworks.git`, tag `8.15.0`
> Git download
> Git download
$ /usr/bin/git clone https://github.com/invertase/firestore-ios-sdk-frameworks.git /var/folders/cq/1h1lq3hj5dndl330zc9xn80m0000gp/T/d20230814-46222-nta7zq --template= --single-branch --depth 1 --branch 8.15.0
FirebaseFirestore/AutodetectLeveldb: subspec would include leveldb if used directly or by default.
> Copying FirebaseFirestore from `/Users/punaime/Library/Caches/CocoaPods/Pods/External/FirebaseFirestore/e4fb610867400ab6d3feb96b8d6fe477` to `Pods/FirebaseFirestore`
-> Fetching podspec for `Flutter` from `Flutter`
-> Fetching podspec for `cloud_firestore` from `.symlinks/plugins/cloud_firestore/ios`
cloud_firestore: Using Firebase SDK version '10.12.0' defined in 'firebase_core'
-> Fetching podspec for `firebase_core` from `.symlinks/plugins/firebase_core/ios`
firebase_core: Using Firebase SDK version '10.12.0' defined in 'firebase_core'
Resolving dependencies of `Podfile`
CDN: trunk Relative path: CocoaPods-version.yml exists! Returning local because checking is only performed in repo update
[!] CocoaPods could not find compatible versions for pod "FirebaseFirestore":
In snapshot (Podfile.lock):
FirebaseFirestore (= 10.12.0, ~> 10.12.0)
In Podfile:
FirebaseFirestore (from `https://github.com/invertase/firestore-ios-sdk-frameworks.git`, tag `8.15.0`)
None of your spec sources contain a spec satisfying the dependencies: `FirebaseFirestore (from `https://github.com/invertase/firestore-ios-sdk-frameworks.git`, tag `8.15.0`), FirebaseFirestore (= 10.12.0, ~> 10.12.0)`.
You have either:
* out-of-date source repos which you can update with `pod repo update` or with `pod install --repo-update`.
* mistyped the name or version.
* not added the source repo that hosts the Podspec to your Podfile.
(以下省略)
ここまでで、iOS、Androidともにエラー無くビルドできることを確認してみてください。
Cloud Firestore パッケージを使用するには、cloud_firestore.dart
をインポートする必要があります。
import 'package:cloud_firestore/cloud_firestore.dart';
次にmain.dart
ファイルで、Firestore インスタンスを読み込みます。_MyHomePageState
クラスにFirebaseFirestore
のインスタンスを生成してdb
に格納します。このようにすることで、Firestoreのデータにアクセスする準備が整います。
FirebaseFirestore db = FirebaseFirestore.instance;
(変更前)
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
(変更後)
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
db = FirebaseFirestore.instance; //追加
FlatterでのCloud Firestoreの基本設定は、以上となります。次回は、Firestore側の設定を行います。