Container
はFlutterで使われる基本的なウィジェットです。シンプルに言うと、それはスタイル付きのボックスを表します。このボックスの中に、テキストや画像などの別のウィジェットを配置することができます。また、サイズや色、余白などの様々な属性を使って見た目を調整することができます。
Container({
Key? key,
this.alignment,
this.padding,
Color? color,
Decoration? decoration,
this.margin,
this.width,
this.height,
... // その他の属性
this.child,
})
説明
child
: Containerの中に配置するウィジェット。例えば、TextやIconなど。padding
: 内部の余白。子ウィジェットの周りのスペース。color
: 背景色。decoration
: 複雑な装飾。例:ボーダー、グラデーション、シャドウなど。margin
: 外部の余白。Containerの周りのスペース。width & height
: Containerのサイズ。
- デザイン要素: 背景色やボーダーを持つボタンやカードとして。
- スペース調整: 他のウィジェットとの間隔を取るため。
- グルーピング: 他のウィジェットを一つのグループとしてまとめる時。
Container(
color: Colors.blue,
child: const Text('これは青い背景のテキストです'),
),

Container(
padding: EdgeInsets.all(20.0),
margin: EdgeInsets.symmetric(horizontal: 30.0),
decoration: BoxDecoration(
border: Border.all(color: Colors.red, width: 2.0),
),
child: const Text('これはボーダー付きのテキストです'),
),
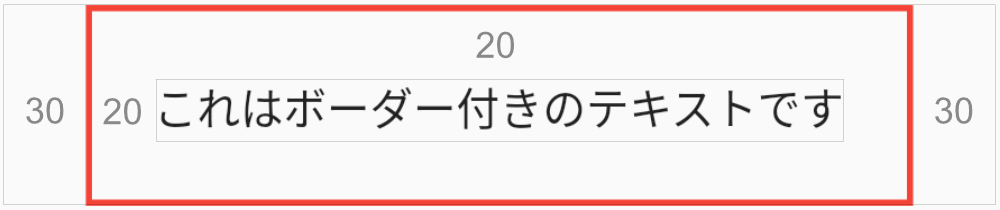
すべての辺の余白を同じ幅にする。
margin: EdgeInsets.all(20.0),
左右、上下の余白を同じ幅にする。
margin: EdgeInsets.symmetric(horizontal: 30.0),
margin: EdgeInsets.symmetric(vertical: 30.0),
各辺ごとに余白を指定する。
margin: EdgeInsets.only(top: 10, right: 30, bottom: 30, left: 10)
Container(
padding: const EdgeInsets.all(20.0),
margin: const EdgeInsets.all(10.0),
decoration: BoxDecoration(
color: Colors.green,
borderRadius: BorderRadius.circular(15.0),
),
child: const Text('角が丸い緑の背景のテキスト'),
),
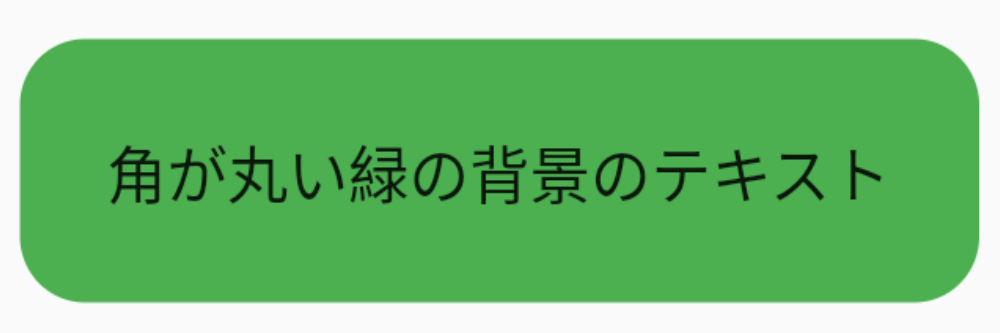
Container(
width: 200,
height: 100,
decoration: const BoxDecoration(
gradient: LinearGradient(
colors: [Colors.green, Colors.greenAccent],
begin: Alignment.topLeft,
end: Alignment.bottomRight,
),
),
),
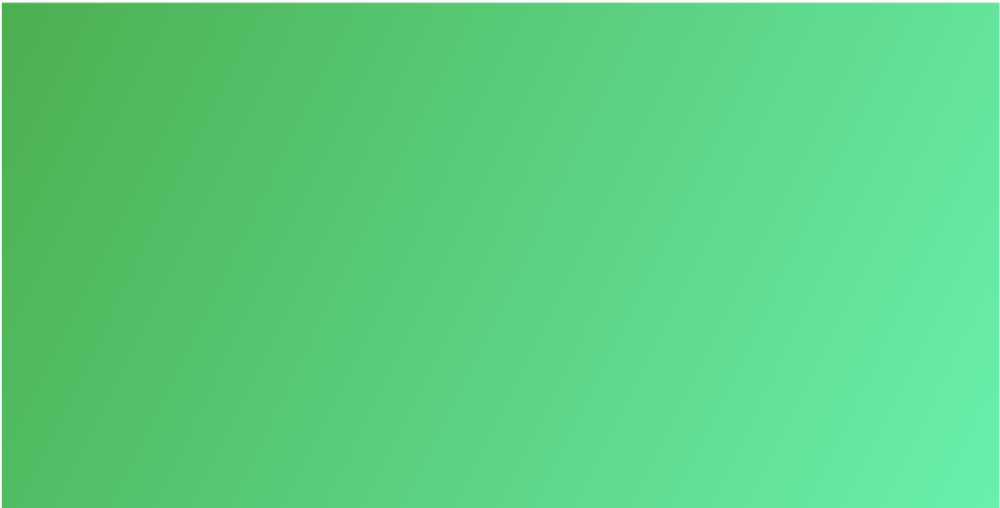
Container(
width: 300.0,
child: AspectRatio(
aspectRatio: 16/9,
child: Container(
color: Colors.green,
child: const Text('16:9のアスペクト比を持つコンテナ'),
),
),
),
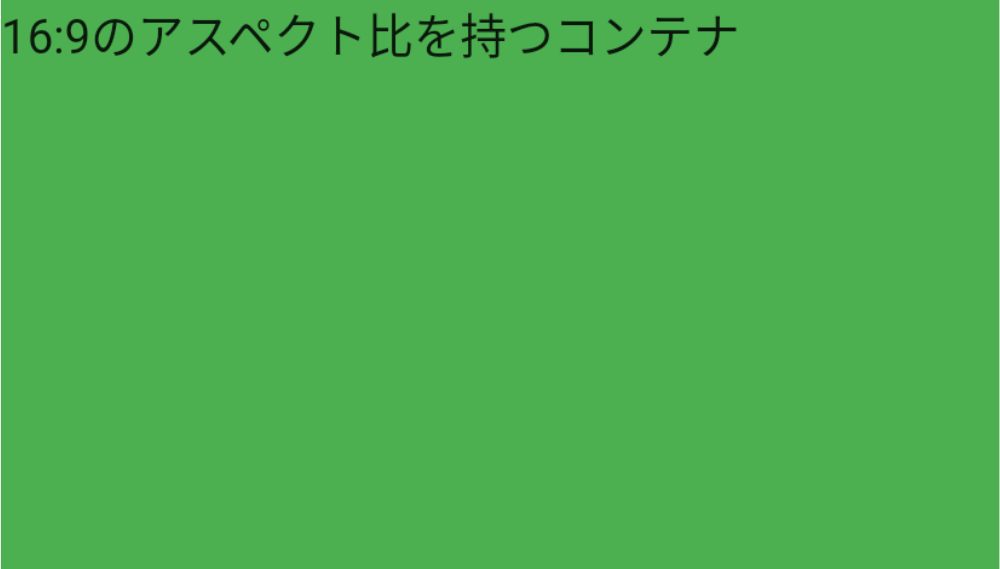